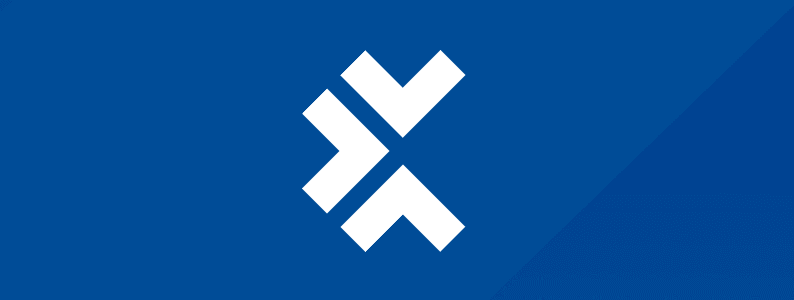
Efficient test automation is crucial for maintaining code quality and accelerating software development cycles. Cucumber offers a unique approach to test automation that embraces collaboration and clarity. This article explores what Cucumber is, its benefits, and how you can get started with it to enhance your test automation workflow.
What is cucumber?
Cucumber is an open-source testing framework that supports behavior-driven development (BDD). You can use it to create automated tests that are easy to understand and maintain. As creator Aslak Hellesøy put it, “Cucumber is a tool that supports Behavior-Driven Development (BDD). It offers a way to write tests that anybody can understand, regardless of their technical knowledge.” Developers and testers can write tests in plain language using Gherkin syntax, making them understandable for both technical teams and nontechnical stakeholders while being executable by automated systems.
How does cucumber work?
Cucumber tests are composed of five main components:
- Gherkin language: Gherkin is a domain-specific language for writing Cucumber tests. It uses simple and clear syntax to define test scenarios in a given-when-then format.
-
- Given: Describes the initial context or state.
- When: Describes the action or event that triggers a behavior.
- Then: Describes the expected outcome.
- Feature files: These files contain user stories and scenarios written in Gherkin. Each feature file represents a specific functionality.
- Step definitions: Step definitions are written in a programming language (e.g., Ruby, Java, JavaScript) and provide the implementation for each step described in the feature files. This maps the steps written in Gherkin to code.
- Test execution: Cucumber runs the tests by executing the step definitions in the context of the feature files. It compares the actual behavior of the application with the expected behavior and reports the results.
- Hooks: Hooks are used to set up and tear down test scenarios. They allow you to perform actions before and after tests, such as initializing test data and cleaning up resources.
Features of Cucumber
- Readable syntax: Cucumber uses Gherkin, a domain-specific language that makes tests readable and understandable by anyone involved in the project.
- Integration with various languages: Cucumber supports multiple programming languages, making it flexible for different tech stacks.
- Automatic test execution: Once written, Cucumber tests are automatically executable, providing continuous integration and testing.
- Support for multiple platforms: Cucumber integrates with tools like Selenium and Appium for web and mobile testing respectively.
Benefits of using cucumber testing
- Enhanced collaboration: Cucumber’s use of Gherkin, a domain-specific language, enables clear communication between technical and nontechnical team members. This ensures that all stakeholders have a shared understanding of the software’s expected behavior.
- Readable test cases: Tests are written in a language that’s easy to understand, which improves the maintainability of the test suite and makes it easier to update tests as requirements change.
- Automated testing: Cucumber integrates with various testing tools and frameworks (e.g., Selenium, Cypress) to automate tests, which helps identify issues early in the development cycle.
- Behavior-driven development (BDD): Cucumber supports BDD, allowing teams to write tests based on user stories and business requirements, aligning testing closely with business goals.
Cucumber supports BDD, allowing teams to write tests in a language that’s accessible to everyone involved in the project.
BDD in cucumber test automation
Behavior-driven development encourages collaboration between developers, QA, and nontechnical stakeholders. Cucumber supports BDD by allowing teams to write tests in a language that’s accessible to everyone involved in the project. This approach helps ensure that the software meets the business requirements and provides a clear understanding of the application’s expected behavior.
Benefits of BDD in cucumber framework
- Clear requirements: BDD helps clarify and refine requirements by discussing and documenting them in the form of scenarios.
- Increased transparency: By involving all stakeholders in the test creation process, BDD promotes transparency and ensures that everyone has a shared understanding of the application’s functionality.
- Faster feedback: BDD allows for quicker identification of issues, as the tests are written in collaboration with stakeholders who understand business needs.
Limitations of behavior-driven development
While BDD and Cucumber offer numerous advantages, there are some limitations:
- Initial learning curve: Teams new to BDD may face a learning curve when adopting the methodology and tools.
- Test maintenance: As the application evolves, maintaining BDD scenarios and keeping them aligned with the current requirements can be challenging.
- Overhead: Implementing BDD can introduce additional overhead in terms of time and resources required for writing and maintaining feature files.
Getting Started With Cucumber
To get started with Cucumber in Python, you’ll need to install the following:
- Python (3.6 or later)
- Behave (Cucumber for Python)
- Selenium (for web testing, if needed)
Install these dependencies using pip:
pip install behave selenium
Writing your first cucumber test
Let’s create a simple Cucumber test for a calculator application.
Step 1: Create a Feature File
Create a file named calculator.feature:
Feature: Calculator
As a user
I want to perform basic arithmetic operations
So that I can do quick calculations
Scenario: Add two numbers
Given I have a calculator
When I add 5 and 3
Then the result should be 8
Step 2: Implement Step Definitions
Create a file named
calculator_steps.py:
from behave import given, when, then
from calculator import Calculator
@given('I have a calculator')
def step_impl(context):
context.calculator = Calculator()
@when('I add {num1:d} and {num2:d}')
def step_impl(context, num1, num2):
context.result = context.calculator.add(num1, num2)
@then('the result should be {expected:d}')
def step_impl(context, expected):
assert context.result == expected, f"Expected {expected}, but got {context.result}"
Step 3: Implement the Calculator Class
Create a file named
calculator.py
in your project root:
class Calculator:
def add(self, a, b):
return a + b
Step 4: Run the Test
Run the test using the following command:
behave
You should see an output indicating that the test has passed.
Cucumber offers several advanced features to enhance your test automation
Advanced cucumber features
Cucumber offers several advanced features to enhance your test automation:
1.Scenario outlines: This allows you to run the same scenario with multiple sets of data.
Scenario Outline: Add two numbers
Given I have a calculator
When I add <num1> and <num2>
Then the result should be <result>
Examples:
| num1 | num2 | result |
| 5 | 3 | 8 |
| 2 | 7 | 9 |
| 10 | -3 | 7 |
2.Tags: Tags in Cucumber are used to organize and selectively run tests. They’re useful for categorizing scenarios and features, allowing you to run specific subsets of your test suite.
Here’s an example of how to use tags in a feature file:
@calculator
Feature: Calculator Operations
@addition
Scenario: Add two numbers
Given I have a calculator
When I add 5 and 3
Then the result should be 8
@subtraction
Scenario: Subtract two numbers
Given I have a calculator
When I subtract 7 from 10
Then the result should be 3
@multiplication @slow
Scenario: Multiply two numbers
Given I have a calculator
When I multiply 6 and 4
Then the result should be 24
- @calculator is a tag for the entire feature.
- @addition, @subtraction, and @multiplication are tags for individual scenarios.
- @slow is an additional tag that might indicate a longer-running test.
You can run specific tags using the command line.
# Run all scenarios with the 'addition' tag
Here’s an example
environment.py
file demonstrating various types of hooks:
from selenium import webdriver
def before_all(context):
print("Starting the test suite")
context.config.setup_logging()
def after_all(context):
print("All tests completed")
def before_feature(context, feature):
if 'web' in feature.tags:
context.browser = webdriver.Chrome()
context.browser.implicitly_wait(10)
def after_feature(context, feature):
if 'web' in feature.tags:
context.browser.quit()
def before_scenario(context, scenario):
if 'db' in scenario.tags:
context.db = connect_to_database()
def after_scenario(context, scenario):
if 'db' in scenario.tags:
context.db.close()
def before_step(context, step):
print(f"Starting step: {step.name}")
def after_step(context, step):
if step.status == "failed":
print(f"Step failed: {step.name}")
# You could add code here to take a screenshot, for example
def before_tag(context, tag):
if tag == "slow":
context.config.setup_slowtest_logging()
def after_tag(context, tag):
if tag == "slow":
context.config.teardown_slowtest_logging()
andbefore_all
run before and after the entire test suite.after_all
andbefore_feature
run before and after each feature.after_feature
andbefore_scenario
run before and after each scenario.after_scenario
andbefore_step
run before and after each step.after_step
andbefore_tag
run before and after scenarios with specific tags.after_tag
These hooks allow you to set up and tear down resources, initialize test environments, handle logging, and perform other setup and cleanup tasks as needed throughout your test execution.
For example, the
before_feature
hook checks if the feature has a ‘web’ tag, and if so, it initializes a Selenium WebDriver. The corresponding after_feature
hook then closes the browser after the feature is complete.
4.Reporting: Cucumber generates reports that provide detailed information about test execution. While the default console output is useful, you can enhance your reporting with additional tools.
For Python and Behave, you can use plugins like behave-html-formatter to generate HTML reports:
pip install behave-html-formatter behave -f html -o report.html
This will generate an HTML report with detailed test results, including passed and failed scenarios, step definitions, and execution times.
5.Parameterized Steps: Parameterized steps allow you to reuse step definitions with different inputs. Here’s an example:
Scenario: Verify user registration
Given there is a user "John" with email "john@example.com"
When "John" tries to register with email "john@example.com"
Then the registration should fail
Scenario: Successful user registration
Given there is no user with email "jane@example.com"
When "Jane" tries to register with email "jane@example.com"
Then the registration should succeed
The step definitions might look like this:
@given('there is a user "{name}" with email "{email}"')
def step_impl(context, name, email):
# Code to add user to the system
@given('there is no user with email "{email}"')
def step_impl(context, email):
# Code to ensure no user exists with the given email
@when('"{name}" tries to register with email "{email}"')
def step_impl(context, name, email):
context.registration_result = register_user(name, email)
@then('the registration should {result}')
def step_impl(context, result):
expected = result == "succeed"
assert context.registration_result == expected
Best practices for cucumber test automation
- Keep scenarios focused and concise.
- Use declarative rather than imperative style in Gherkin.
- Maintain a clear separation between test logic and step definitions.
- Reuse step definitions across scenarios.
- Use tags to organize and categorize tests.
- Implement proper error handling and reporting.
Conclusion: cucumber test automation
Remember that while Cucumber can be very beneficial, it’s important to use it judiciously. Not all tests need to use Gherkin. For example, you’ll often want to express unit tests directly in code. Use Cucumber where its benefits of readability and collaboration are most valuable, typically for acceptance tests and end-to-end scenarios.
This post was written by Wisdom Ekpotu. Wisdom is a Software & Technical Writer based in Nigeria. Wisdom is passionate about web/mobile technologies, open-source, and building communities. He also helps companies improve the quality of their Technical Documentation.