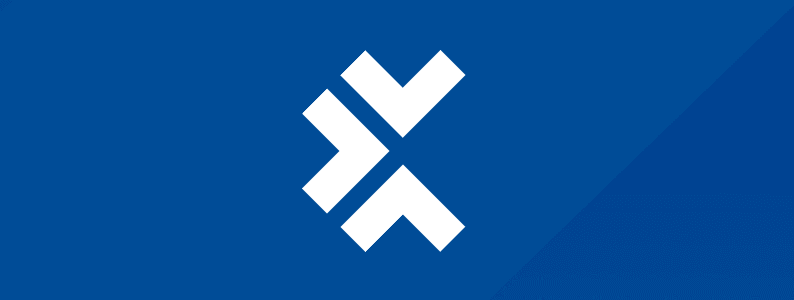
In the rapidly evolving world of web development, ensuring the reliability and performance of web applications is paramount. Automated testing has become a crucial component of the development lifecycle, aiming to catch issues early and ensure that applications work seamlessly across different environments and browsers. Selenium, a powerful tool for automating web browsers, stands out in this domain, offering robust capabilities for testing web applications.
However, when it comes to PHP—a server-side scripting language extensively used for web development—integrating automated testing tools like Selenium might initially seem daunting. PHP developers might question how they can leverage Selenium for their web applications and the benefits of such integration.
This post aims to demystify the integration of Selenium with PHP, providing a comprehensive guide on why this combination is beneficial and how to set it up, and walking through practical examples to get you started. Whether you are a seasoned PHP developer looking to enhance the quality of your web applications or just stepping into the world of automated browser testing, this guide is tailored to help you grasp the basics and understand the advantages of using Selenium with PHP. So, let’s dive in and explore the synergy of Selenium and PHP, paving the way for more reliable, efficient, and robust web application development.
Introduction to Selenium
Selenium is a powerful and versatile framework for automating web browsers, allowing developers and testers to simulate user interactions with web applications. By automating browser actions, Selenium helps ensure that web applications function as expected, leading to a more reliable and robust user experience. Selenium supports various programming languages, including Java, C#, Python, and Ruby, making it accessible to many developers.
Integrating Selenium with PHP
While Selenium does not natively support PHP, it doesn’t mean you are left out in the cold. Third-party libraries and bindings such as Facebook’s WebDriver allow PHP to interact seamlessly with the Selenium WebDriver. This opens up the possibility for PHP developers to leverage the power of Selenium for their web application testing needs.
When it comes to automated testing with Selenium, PHP carries several benefits.
Why use PHP with Selenium?
PHP is widely known for its simplicity, speed, and efficiency, making it a popular choice for web development. When it comes to automated testing with Selenium, PHP carries several benefits:
- Familiarity: For developers already working in a PHP environment, using PHP for automated testing with Selenium means not having to switch contexts or learn a new programming language.
- Integration: PHP can be easily integrated with other tools and services, making it a flexible option for setting up comprehensive testing environments.
- Community support: PHP boasts a large and active community, which means a wealth of resources, libraries, and tools are readily available to help solve any challenges that might arise.
- Efficiency: PHP’s lightweight nature means tests can run quickly and efficiently, ensuring a faster feedback loop during development.
Getting started with PHP and Selenium
Embarking on integrating Selenium with PHP requires a systematic approach to setting up your environment. Ensuring that you have all the necessary tools and dependencies in place is crucial for a smooth and successful implementation. Here’s a step-by-step guide to help you establish a strong foundation:
1. Install Selenium WebDriver
The Selenium WebDriver is the heart of Selenium, providing a programming interface to write and execute test scripts. To get started, you must download and install the WebDriver for the browser you intend to automate. The most common browsers supported are Chrome, Firefox, and Edge.
- Chrome: Download the ChromeDriver from the official website.
- Firefox: Download the GeckoDriver from the official website.
- Edge: Download the EdgeDriver from the official website.
Make sure to place the WebDriver executable in a directory in your system’s PATH or provide the path to the executable in your test scripts.
2. Install a PHP interpreter
You must have PHP installed on your machine to write and run PHP scripts. Download and install the latest version of PHP from the official PHP website. Verify the installation by running php -v in your terminal or command prompt, which should display the installed PHP version.
3. Install Composer
Composer is a dependency manager for PHP, simplifying the process of managing libraries and tools in your project. Install Composer by following the instructions provided on the official Composer website. Once installed, you can use Composer to install libraries, manage autoloaders, and handle project dependencies.
4. Install Facebook’s WebDriver
Facebook’s WebDriver is a PHP library that provides an interface to interact with the Selenium WebDriver. You can install it via Composer by running the following command in your project directory:
composer require facebook/webdriver
This command will download and install the WebDriver library, making it available in your PHP scripts.
5. Verify your set-up
To ensure you have everything set up correctly, create a simple PHP script to open a browser and navigate to a web page. Here’s an example script:
<?php
require_once(‘vendor/autoload.php’);
use Facebook\WebDriver\Remote\DesiredCapabilities;
use Facebook\WebDriver\Remote\RemoteWebDriver;
$host = ‘http://localhost:4444/wd/hub‘; // this is the default Selenium server URL
$capabilities = DesiredCapabilities::chrome();
$driver = RemoteWebDriver::create($host, $capabilities);
$driver->get(‘https://www.example.com’);
echo “Browser opened successfully!”;
$driver->quit();
?>
Run the script using PHP from the command line:
php your_script_name.php
If everything is set up correctly, this script will open your browser, navigate to https://www.example.com, and close the browser.
By following these detailed steps, you have created a solid foundation for integrating Selenium with PHP, paving the way for writing and executing comprehensive automated tests for your web applications. With your environment ready, you can start exploring the capabilities of Selenium and PHP, creating automated tests to ensure the reliability and performance of your web applications.
Start exploring the capabilities of Selenium and PHP, creating automated tests to ensure the reliability and performance of your web applications.
Writing your first test
Now that your environment is ready, let’s walk through an example of a basic test:
- Create a test file: Create a new PHP file for your test, for example, test.php.
- Write your test: Open your test file and write the following code to test a login functionality:
<?php
require_once(‘vendor/autoload.php’);
use Facebook\WebDriver\Remote\DesiredCapabilities;
use Facebook\WebDriver\Remote\RemoteWebDriver;
use Facebook\WebDriver\WebDriverBy;
// Start a browser session
$host = ‘http://localhost:4444/wd/hub‘; // this is the default
$capabilities = DesiredCapabilities::chrome();
$driver = RemoteWebDriver::create($host, $capabilities);
// Navigate to the login page
$driver->get(‘https://example.com/login’);
// Enter username and password
$driver->findElement(WebDriverBy::id(‘username’))->sendKeys(‘my_username’);
$driver->findElement(WebDriverBy::id(‘password’))->sendKeys(‘my_password’);
// Click the login button
$driver->findElement(WebDriverBy::id(‘login_button’))->click();
// Assert the login was successful
$welcomeMessage = $driver->findElement(WebDriverBy::id(‘welcome_message’))->getText();
if ($welcomeMessage != ‘Welcome, my_username!’) {
throw new Exception(‘Login failed!’);
}
// Close the browser session
$driver->quit();
echo “Test passed successfully!”;
?>
This script opens a browser, navigates to a login page, enters a username and password, clicks the login button, and then checks to see whether the login was successful.
Selenium + PHP best practices and troubleshooting
- Keep your tests DRY (don’t repeat yourself): Utilize functions and classes to reuse code across different tests.
- Handle waits properly: Implement proper wait strategies to deal with asynchronous operations.
- Keep tests isolated: Each test should be independent of others, ensuring a clean test environment.
- Use descriptive assertions: Make your assertion messages as descriptive as possible, so it’s clear what went wrong if a test fails.
- Check console logs: If a test fails, check the browser’s console logs for errors or warnings.
- Update WebDriver regularly: Ensure that your WebDriver is always up to date with the browser version you are testing on.
By following these steps and best practices, PHP developers can effectively leverage Selenium for automated web testing. This way you can bring a new level of reliability and efficiency to their development workflow. Be sure to check out more resources and tutorials on Tricentis ShiftSync to deepen your understanding and skills in automated testing with Selenium and PHP.
This post was written by Juan Reyes. As an entrepreneur, skilled engineer, and mental health champion, Juan pursues sustainable self-growth, embodying leadership, wit, and passion. With over 15 years of experience in the tech industry, Juan has had the opportunity to work with some of the most prominent players in mobile development, web development, and e-commerce in Japan and the US. Juan’s Github you may find here.